我们经常在一些电商平台上注册的时候,都会需要进行邮箱验证,对于php程序开发工程师需要弄明白其中的实现原理和流程
1,先去qq邮箱中的 点击设置-》点击帐户-》将smtp的权限开启 如图:
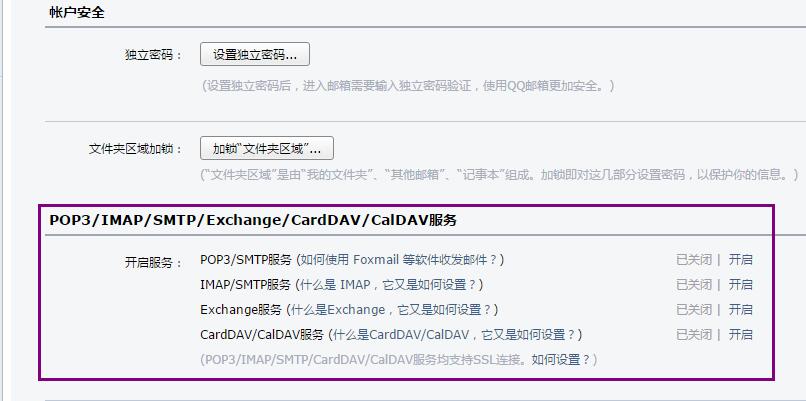
如果是设置POP3和SMTP的SSL加密方式,则端口如下:
POP3服务器(端口995)
SMTP服务器(端口465或587)。
2、查看你的Openssl和Socketsd是否支持:php -m查看
利用OpenSSL库对Socket传输进行安全加密。
3、表单部分
<form id="reg" action="register.php" method="post">
<p>用户名:<input type="text" class="input" name="username" id="user"></p>
<p>密 码:<input type="password" class="input" name="password" id="pass"></p>
<p>E-mail:<input type="text" class="input" name="email" id="email"></p>
<p><input type="submit" class="btn" value="提交注册"></p>
</form>
4、表单提交部分
<!DOCTYPE HTML>
<html>
<head>
<meta charset="utf-8">
<title>PHP用户注册邮箱验证激活帐号</title>
<style type="text/css">
.demo {margin: 20px auto; width: 400px; border: 1px solid #ccc; line-height: 50px;text-align: center;}
.input {width: 150px; height: 25px; border: 1px solid #ccc;}
.btn {padding: 5px 15px; font-size: 16px; font-family: '微软雅黑'; background:#ff0066; color: #fff; border: none;}
</style>
<script type="text/javascript">
function chk_form(){
var user = document.getElementById("user");
if(user.value==""){
alert("用户名不能为空!");
return false;
//user.focus();
}
var pass = document.getElementById("pass");
if(pass.value==""){
alert("密码不能为空!");
return false;
//pass.focus();
}
var email = document.getElementById("email");
if(email.value==""){
alert("Email不能为空!");
return false;
//email.focus();
}
var preg = /^\w+([-+.]\w+)*@\w+([-.]\w+)*\.\w+([-.]\w+)*/; //匹配Email
if(!preg.test(email.value)){
alert("Email格式错误!");
return false;
//email.focus();
}
}
</script>
</head>
<body>
<div id="main">
<div class="demo">
<form id="reg" action="register.php" method="post" onsubmit="return chk_form();">
用户名:<input type="text" class="input" name="username" id="user"><br>
密 码:<input type="password" class="input" name="password" id="pass"><br>
邮 箱:<input type="text" class="input" name="email" id="email"><br>
<input type="submit" class="btn" value="提交注册">
</form>
</div>
</div>
</body>
<html>
5,如果注册成功,发送邮箱验证码,这里负责发送。
register.php部分
<?php
include_once("connect.php");//连接数据库
// include_once("smtp.class.php");//邮件发送类
$username = stripslashes(trim($_POST['username']));
$sql ="select id from t_user where username='{$username}'";
// $query = mysqli_query("'");
$res = mysqli_query($link, $sql);
if($res && mysqli_num_rows($res)>0){
echo '用户名已存在,请换个其他的用户名';
exit;
}
//构造激活码
$password = md5(trim($_POST['password'])); //加密密码
$email = trim($_POST['email']); //邮箱
$regtime = time();
$token = md5($username.$password.$regtime); //创建用于激活识别码
$token_exptime = time()+60*60*24;//过期时间为24小时后
$sql = "insert into `t_user` (`username`,`password`,`email`,`token`,`token_exptime`,`regtime`)
values ('$username','$password','$email','$token','$token_exptime','$regtime')";
// echo $sql;
$res1 = mysqli_query($link, $sql);
if(mysqli_insert_id($link)){
//邮件发送
require 'class.phpmailer.php';
require 'class.smtp.php';
$mail = new PHPMailer;
//$mail->SMTPDebug = 3; // Enable verbose debug output
$mail->isSMTP(); // Set mailer to use SMTP
$mail->Host = 'smtp.qq.com'; // Specify main and backup SMTP servers
$mail->SMTPAuth = true; // Enable SMTP authentication
$mail->Username = '1391241655@qq.com'; // SMTP username
$mail->Password = 'dzrxckopdnxuhjhf'; // SMTP password
$mail->SMTPSecure = 'ssl'; // Enable TLS encryption, `ssl` also accepted
$mail->Port = 465; // TCP port to connect to
$mail->setFrom('1391241655@qq.com', '发件人');
$email = $_POST['email'];
$mail->addAddress($email, '.'); // Add a recipient
// Name is optional
$mail->addReplyTo($email, 'php');
//$mail->addCC('cc@example.com');
//$mail->addBCC('bcc@example.com');
$mail->isHTML(true); // Set email format to HTML
$mail->Subject = '标题';
$mail->Body = "发送的内容";
// $mail->AltBody = '发送的内容22';
if(!$mail->send()) {
//输出错误信息
echo 'Mailer Error: ' . $mail->ErrorInfo;
return false;
} else {
echo 'Message has been sent'; //成功输出
return true;
}
}
?>
6、验证部分
include_once("connect.php");//连接数据库
$verify = stripslashes(trim($_GET['verify']));
$nowtime = time();
$sql = "select id,token_exptime from t_user where status='0' and `token`='$verify'";
$res= mysql_query($link, $sql);
$row = mysql_fetch_array($res);
if($row){
if($nowtime>$row['token_exptime']){ //24hour
$msg = '您的激活有效期已过,请登录您的帐号重新发送激活邮件.';
}else{
$sql1 ="update t_user set status=1 where id=".$row['id']";
$res1 = mysqli_query($link, $sql1);
if(mysqli_affected_rows($link)!=1) die(0);
$msg = '激活成功!';
}
}else{
$msg = 'error.';
}
echo $msg;